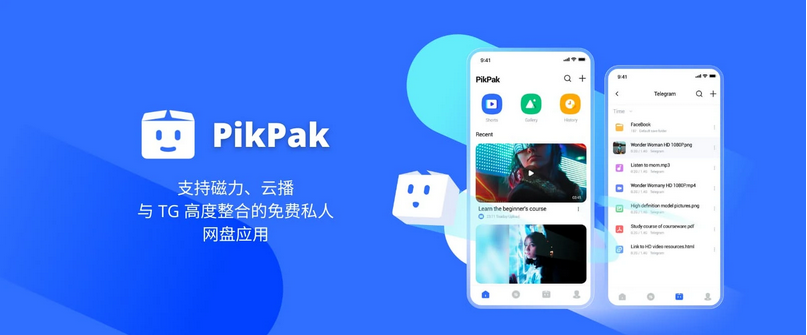
[Linux操作系统]深入探索Linux系统编程实践|linux编程实践教程,Linux系统编程实践
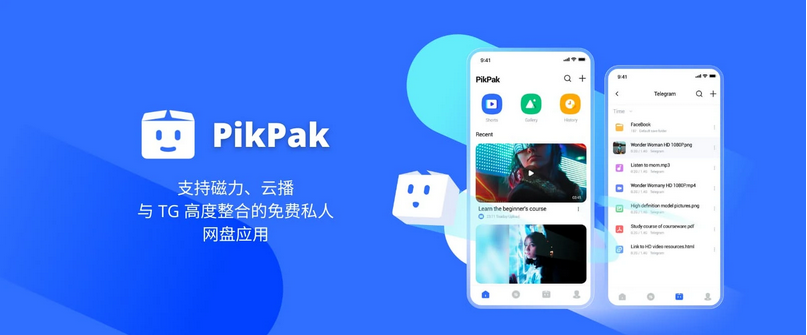
本书深入探讨了Linux系统编程的核心技术和实践方法。通过详细的教程和实例,读者可掌握Linux环境下的编程技巧,包括进程管理、文件操作、网络通信等关键领域。该书适合有一定编程基础的读者,旨在提升其在Linux系统上的开发能力,是Linux编程实践的理想指南。通过学习,读者将能更高效地利用Linux系统资源,编写出高效、稳定的系统级应用程序。
本文目录导读:
Linux系统作为开源操作系统的代表,以其稳定性和灵活性在服务器、嵌入式系统等领域得到了广泛应用,对于开发者而言,掌握Linux系统编程不仅是提升技能的必经之路,更是深入理解操作系统原理的重要途径,本文将围绕Linux系统编程实践,探讨其核心概念、常用技术以及实际应用案例。
Linux系统编程概述
Linux系统编程主要涉及与操作系统内核的交互,包括进程管理、内存管理、文件系统操作、网络通信等方面,与普通的应用程序开发不同,系统编程更注重资源的有效利用和系统性能的优化。
1、进程管理:进程是Linux系统中的基本执行单元,系统编程中常见的进程管理操作包括进程创建(fork)、执行新程序(exec)、进程间通信(IPC)等。
2、内存管理:Linux提供了丰富的内存管理机制,如动态内存分配(malloc、free)、内存映射(mmap)等。
3、文件系统操作:文件系统是Linux存储数据的核心部分,系统编程中常涉及文件的打开、读取、写入、关闭等操作。
4、网络通信:Linux支持多种网络协议,系统编程中常见的网络操作包括套接字编程、网络协议栈的使用等。
核心技术与实践
1. 进程管理实践
进程创建与执行
在Linux中,创建新进程通常使用fork
系统调用。fork
会创建一个与父进程几乎完全相同的子进程,子进程可以通过exec
系列函数执行新的程序。
#include <stdio.h> #include <unistd.h> #include <sys/types.h> int main() { pid_t pid = fork(); if (pid == 0) { // 子进程 execlp("ls", "ls", "-a", NULL); } else if (pid > 0) { // 父进程 printf("子进程PID: %d ", pid); } else { // fork失败 perror("fork"); } return 0; }
进程间通信
进程间通信(IPC)是系统编程中的重要内容,常见的IPC机制包括管道(pipe)、消息队列、共享内存、信号量等。
#include <stdio.h> #include <unistd.h> int main() { int pipefd[2]; if (pipe(pipefd) == -1) { perror("pipe"); return 1; } pid_t pid = fork(); if (pid == 0) { // 子进程,写数据 close(pipefd[0]); // 关闭读端 write(pipefd[1], "Hello, Parent!", 14); close(pipefd[1]); } else if (pid > 0) { // 父进程,读数据 close(pipefd[1]); // 关闭写端 char buffer[20]; read(pipefd[0], buffer, 20); printf("收到子进程的消息: %s ", buffer); close(pipefd[0]); } else { perror("fork"); } return 0; }
2. 内存管理实践
动态内存分配
动态内存分配是系统编程中常见的操作,常用的函数包括malloc
、free
、calloc
和realloc
。
#include <stdio.h> #include <stdlib.h> int main() { int *arr = (int *)malloc(10 * sizeof(int)); if (arr == NULL) { perror("malloc"); return 1; } for (int i = 0; i < 10; i++) { arr[i] = i; } for (int i = 0; i < 10; i++) { printf("%d ", arr[i]); } printf(" "); free(arr); return 0; }
内存映射
内存映射是一种将文件或设备的内容映射到进程地址空间的技术,常用于高效文件操作。
#include <stdio.h> #include <stdlib.h> #include <fcntl.h> #include <sys/mman.h> #include <sys/stat.h> #include <unistd.h> int main() { const char *file_path = "example.txt"; int fd = open(file_path, O_RDONLY); if (fd == -1) { perror("open"); return 1; } struct stat sb; if (fstat(fd, &sb) == -1) { perror("fstat"); close(fd); return 1; } char *map = mmap(NULL, sb.st_size, PROT_READ, MAP_PRIVATE, fd, 0); if (map == MAP_FAILED) { perror("mmap"); close(fd); return 1; } for (size_t i = 0; i < sb.st_size; i++) { putchar(map[i]); } munmap(map, sb.st_size); close(fd); return 0; }
3. 文件系统操作实践
文件基本操作
文件操作是系统编程中的基础内容,包括文件的打开、读取、写入和关闭。
#include <stdio.h> #include <stdlib.h> #include <fcntl.h> #include <unistd.h> int main() { const char *file_path = "output.txt"; int fd = open(file_path, O_WRONLY | O_CREAT | O_TRUNC, 0644); if (fd == -1) { perror("open"); return 1; } const char *data = "Hello, World!"; if (write(fd, data, 13) == -1) { perror("write"); close(fd); return 1; } close(fd); return 0; }
目录操作
目录操作包括创建目录、删除目录、读取目录内容等。
#include <stdio.h> #include <stdlib.h> #include <sys/types.h> #include <sys/stat.h> #include <unistd.h> #include <dirent.h> int main() { const char *dir_path = "new_dir"; if (mkdir(dir_path, 0755) == -1) { perror("mkdir"); return 1; } DIR *dir = opendir(dir_path); if (dir == NULL) { perror("opendir"); rmdir(dir_path); return 1; } struct dirent *entry; while ((entry = readdir(dir)) != NULL) { printf("%s ", entry->d_name); } closedir(dir); if (rmdir(dir_path) == -1) { perror("rmdir"); return 1; } return 0; }
4. 网络通信实践
套接字编程
套接字编程是网络通信的基础,常见的操作包括创建套接字、绑定地址、监听连接、接收和发送数据等。
#include <stdio.h> #include <stdlib.h> #include <string.h> #include <unistd.h> #include <arpa/inet.h> int main() { int server_fd, new_socket; struct sockaddr_in address; int opt = 1; int addrlen = sizeof(address); char buffer[1024] = {0}; const char *hello = "Hello from server"; if ((server_fd = socket(AF_INET, SOCK_STREAM, 0)) == 0) { perror("socket"); exit(EXIT_FAILURE); } if (setsockopt(server_fd, SOL_SOCKET, SO_REUSEADDR | SO_REUSEPORT, &opt, sizeof(opt))) { perror("setsockopt"); exit(EXIT_FAILURE); } address.sin_family = AF_INET; address.sin_addr.s_addr = INADDR_ANY; address.sin_port = htons(8080); if (bind(server_fd, (struct sockaddr *)&address, sizeof(address)) < 0) { perror("bind"); exit(EXIT_FAILURE); } if (listen(server_fd, 3) < 0) { perror("listen"); exit(EXIT_FAILURE); } if ((new_socket = accept(server_fd, (struct sockaddr *)&address, (socklen_t*)&addrlen)) < 0) { perror("accept"); exit(EXIT_FAILURE); } read(new_socket, buffer, 1024); printf("%s ", buffer); send(new_socket, hello, strlen(hello), 0); printf("Hello message sent "); close(new_socket); close(server_fd); return 0; }
实际应用案例
案例一:多进程日志系统
在实际应用中,多进程日志系统是一个典型的系统编程案例,通过多个进程协同工作,可以实现高效的日志记录和管理。
// 日志写入进程 #include <stdio.h> #include <unistd.h> #include <sys/types.h> #include <sys/ipc.h> #include <sys/msg.h> struct message { long msg_type; char msg_text[100]; }; int main() { key_t key = ftok("log", 65); int msgid = msgget(key, 0666 | IPC_CREAT); struct message msg; while (1) { msgrcv(msgid





