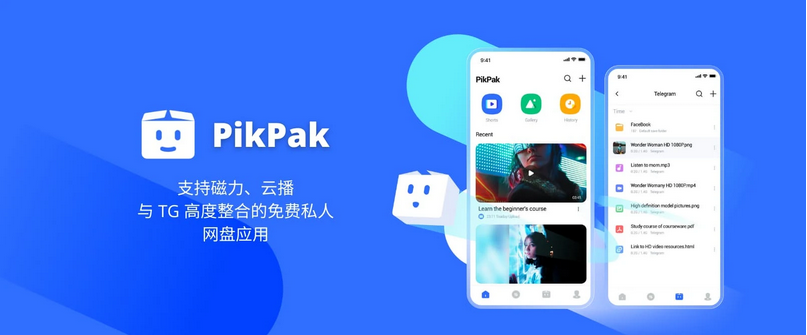
推荐阅读:
[AI-人工智能]免翻墙的AI利器:樱桃茶·智域GPT,让你轻松使用ChatGPT和Midjourney - 免费AIGC工具 - 拼车/合租账号 八折优惠码: AIGCJOEDISCOUNT2024
[AI-人工智能]银河录像局: 国内可靠的AI工具与流媒体的合租平台 高效省钱、现号秒发、翻车赔偿、无限续费|95折优惠码: AIGCJOE
[AI-人工智能]免梯免翻墙-ChatGPT拼车站月卡 | 可用GPT4/GPT4o/o1-preview | 会话隔离 | 全网最低价独享体验ChatGPT/Claude会员服务
[AI-人工智能]边界AICHAT - 超级永久终身会员激活 史诗级神器,口碑炸裂!300万人都在用的AI平台
本书《深入浅出PHP设计模式》旨在通过生动的案例和实用的技巧,帮助开发者提升PHP代码的质量与复用性。书中涵盖了多种PHP设计模式,并针对面试场景提供了相关面试题,助力读者在技术面试中脱颖而出。
本文目录导读:
在软件开发领域,设计模式是一套被反复验证的解决方案,它们能够帮助开发者解决特定场景下的问题,PHP作为一种流行的编程语言,也广泛应用了设计模式来提高代码的复用性、可维护性和可扩展性,本文将详细介绍PHP中的几种常见设计模式,以及如何在项目中合理运用它们。
什么是设计模式
设计模式是一套经过实践检验的、针对特定问题的解决方案,它们通常包含了一组相关的类和对象,这些类和对象之间通过特定的关系组合在一起,以解决特定的问题,设计模式分为三类:创建型、结构型和行为型。
PHP中的创建型设计模式
1、单例模式(Singleton)
单例模式确保一个类只有一个实例,并提供一个全局访问点,在PHP中,单例模式通常用于管理数据库连接、日志记录等。
class Singleton { private static $instance = null; private function __construct() {} public static function getInstance() { if (self::$instance === null) { self::$instance = new self(); } return self::$instance; } private function __clone() {} private function __wakeup() {} }
2、工厂模式(Factory)
工厂模式提供一个创建对象的接口,允许子类决定实例化哪个类,在PHP中,工厂模式常用于创建不同类型的对象。
interface Product { public function operation(); } class ConcreteProductA implements Product { public function operation() { return "Result of ConcreteProductA"; } } class ConcreteProductB implements Product { public function operation() { return "Result of ConcreteProductB"; } } class Factory { public function create($type) { if ($type == "A") { return new ConcreteProductA(); } elseif ($type == "B") { return new ConcreteProductB(); } } }
3、建造者模式(Builder)
建造者模式将一个复杂对象的构建与其表示分离,使得同样的构建过程可以创建不同的表示,在PHP中,建造者模式常用于创建具有多个参数的对象。
class Product { private $partA; private $partB; private $partC; public function setPartA($partA) { $this->partA = $partA; } public function setPartB($partB) { $this->partB = $partB; } public function setPartC($partC) { $this->partC = $partC; } } class Builder { private $product; public function __construct() { $this->product = new Product(); } public function buildPartA() { $this->product->setPartA("PartA"); } public function buildPartB() { $this->product->setPartB("PartB"); } public function buildPartC() { $this->product->setPartC("PartC"); } public function getResult() { return $this->product; } }
PHP中的结构型设计模式
1、适配器模式(Adapter)
适配器模式允许将一个类的接口转换成客户期望的另一个接口,在PHP中,适配器模式常用于兼容不同的接口。
interface Target { public function request(); } class Adaptee { public function specificRequest() { return "specificRequest"; } } class Adapter implements Target { private $adaptee; public function __construct(Adaptee $adaptee) { $this->adaptee = $adaptee; } public function request() { $result = $this->adaptee->specificRequest(); return "Adapter converts " . $result; } }
2、装饰器模式(Decorator)
装饰器模式允许向一个现有的对象添加新的功能,同时又不改变其接口,在PHP中,装饰器模式常用于动态地给对象添加功能。
interface Component { public function operation(); } class ConcreteComponent implements Component { public function operation() { return "ConcreteComponent"; } } class Decorator implements Component { private $component; public function __construct(Component $component) { $this->component = $component; } public function operation() { return $this->component->operation() . " Decorated"; } }
3、桥接模式(Bridge)
桥接模式将抽象部分与实现部分分离,使它们都可以独立地变化,在PHP中,桥接模式常用于实现不同类型的抽象和具体实现之间的组合。
interface Abstraction { public function operation(); } interface Implementor { public function implementation(); } class ConcreteImplementorA implements Implementor { public function implementation() { return "ConcreteImplementorA"; } } class ConcreteImplementorB implements Implementor { public function implementation() { return "ConcreteImplementorB"; } } class RefinedAbstraction extends Abstraction { private $implementor; public function __construct(Implementor $implementor) { $this->implementor = $implementor; } public function operation() { return "RefinedAbstraction(" . $this->implementor->implementation() . ")"; } }
PHP中的行为型设计模式
1、策略模式(Strategy)
策略模式定义了算法家族,分别封装起来,使它们之间可以相互替换,此模式让算法的变化独立于使用算法的客户,在PHP中,策略模式常用于实现不同算法之间的切换。
interface Strategy { public function execute(); } class ConcreteStrategyA implements Strategy { public function execute() { return "ConcreteStrategyA"; } } class ConcreteStrategyB implements Strategy { public function execute() { return "ConcreteStrategyB"; } } class Context { private $strategy; public function __construct(Strategy $strategy) { $this->strategy = $strategy; } public function setStrategy(Strategy $strategy) { $this->strategy = $strategy; } public function executeStrategy() { return $this->strategy->execute(); } }
2、观察者模式(Observer)
观察者模式定义了一种一对多的依赖关系,当一个对象改变状态时,所有依赖于它的对象都会得到通知并自动更新,在PHP中,观察者模式常用于实现事件监听和回调。
interface Observer { public function update(); } interface Subject { public function attach(Observer $observer); public function detach(Observer $observer); public function notify(); } class ConcreteSubject implements Subject { private $observers = []; public function attach(Observer $observer) { $this->observers[] = $observer; } public function detach(Observer $observer) { $index = array_search($observer, $this->observers); if ($index !== false) { unset($this->observers[$index]); } } public function notify() { foreach ($this->observers as $observer) { $observer->update(); } } public function doSomething() { // 改变状态 $this->notify(); } } class ConcreteObserver implements Observer { public function update() { echo "Observer notified "; } }
PHP设计模式为开发者提供了一套成熟的解决方案,通过运用这些设计模式,可以有效地提高代码的复用性、可维护性和可扩展性,在实际项目中,开发者应根据具体需求选择合适的设计模式,并在实践中不断积累经验,以达到更高的代码质量。
关键词:PHP, 设计模式, 单例模式, 工厂模式, 建造者模式, 适配器模式, 装饰器模式, 桥接模式, 策略模式, 观察者模式, 创建型设计模式, 结构型设计模式, 行为型设计模式, 代码复用, 可维护性, 可扩展性, 软件开发, 解决方案, 类, 对象, 接口, 抽象, 实现, 算法, 事件监听, 回调







本文标签属性:
PHP设计模式:php设计模式和代码