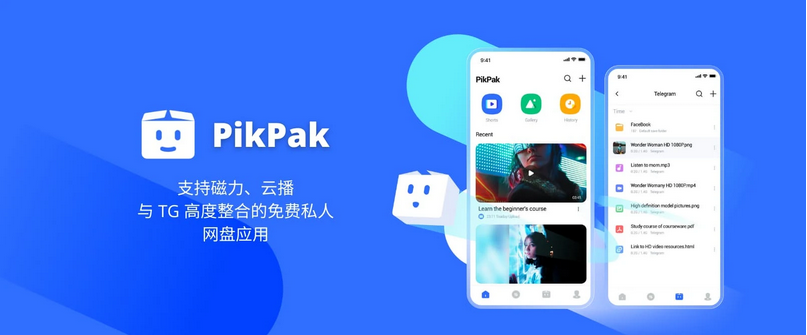
推荐阅读:
[AI-人工智能]免翻墙的AI利器:樱桃茶·智域GPT,让你轻松使用ChatGPT和Midjourney - 免费AIGC工具 - 拼车/合租账号 八折优惠码: AIGCJOEDISCOUNT2024
[AI-人工智能]银河录像局: 国内可靠的AI工具与流媒体的合租平台 高效省钱、现号秒发、翻车赔偿、无限续费|95折优惠码: AIGCJOE
[AI-人工智能]免梯免翻墙-ChatGPT拼车站月卡 | 可用GPT4/GPT4o/o1-preview | 会话隔离 | 全网最低价独享体验ChatGPT/Claude会员服务
[AI-人工智能]边界AICHAT - 超级永久终身会员激活 史诗级神器,口碑炸裂!300万人都在用的AI平台
本文探讨了在Linux操作系统中,PHP处理大文件的策略与实践应用。针对PHP大文件读取和存储的挑战,提出了高效的处理方法。通过优化内存管理、使用流式读取和分块处理技术,有效提升大文件处理性能。结合实际案例,展示了这些策略在实践中的具体应用,为开发者提供了实用的解决方案,确保PHP在处理大文件时的稳定性和效率。
本文目录导读:
在Web开发中,处理大文件是一个常见且具有挑战性的任务,PHP作为一种广泛使用的编程语言,在处理大文件时需要特别关注性能和资源管理,本文将深入探讨PHP大文件处理的策略、技巧以及实践应用,帮助开发者高效应对这一难题。
PHP大文件处理的挑战
1、内存消耗:PHP默认会将整个文件加载到内存中,处理大文件时容易导致内存溢出。
2、执行时间:大文件处理通常需要较长时间,容易触发PHP的最大执行时间限制。
3、I/O性能:频繁的磁盘读写操作会显著影响处理速度。
4、网络传输:如果涉及文件上传或下载,网络延迟和带宽限制也会成为瓶颈。
高效处理策略
1、分块读取:避免一次性加载整个文件,采用分块读取的方式逐步处理。
```php
$file = fopen("largefile.txt", "r");
while (!feof($file)) {
$chunk = fread($file, 1024 * 1024); // 读取1MB大小的数据
// 处理数据
}
fclose($file);
```
2、流式处理:利用PHP的流式处理功能,逐行读取文件。
```php
$file = fopen("largefile.txt", "r");
while ($Line = fgets($file)) {
// 处理每一行数据
}
fclose($file);
```
3、调整PHP配置:增加memory_limit
和max_executiOn_time
的值,以适应大文件处理的需求。
```php
ini_set('memory_limit', '512M');
ini_set('max_execution_time', 300); // 300秒
```
4、使用临时文件:将大文件分割成多个小文件,分别处理后再合并。
```php
$file = fopen("largefile.txt", "r");
$part = 1;
$tempFile = fopen("part{$part}.txt", "w");
while (!feof($file)) {
$chunk = fread($file, 1024 * 1024 * 10); // 读取10MB大小的数据
fwrite($tempFile, $chunk);
fclose($tempFile);
// 处理临时文件
$part++;
$tempFile = fopen("part{$part}.txt", "w");
}
fclose($file);
```
5、异步处理:利用PHP的异步编程库,如ReactPHP,提高处理效率。
```php
$loop = ReactEventLoopFactory::create();
$file = new ReactStreamReadableStream(fopen("largefile.txt", "r"), $loop);
$file->on('data', funCTIon ($chunk) {
// 处理数据
});
$loop->run();
```
实践应用
1、文件上传:处理大文件上传时,可以使用分块上传和断点续传技术。
```php
$chunkSize = 1024 * 1024 * 5; // 5MB
$totalSize = $_POST['totalSize'];
$chunk = file_get_contents('php://input');
$targetFile = "upload_" . $_POST['filename'];
$tempFile = fopen($targetFile, "ab");
fwrite($tempFile, $chunk);
fclose($tempFile);
if (filesize($targetFile) == $totalSize) {
// 文件上传完成
}
```
2、文件下载:使用readfile
或fpassthru
函数实现大文件下载,避免内存溢出。
```php
$file = "largefile.zip";
header('Content-Type: application/octet-stream');
header('Content-Disposition: attachment; filename="' . basename($file) . '"');
header('Content-Length: ' . filesize($file));
readfile($file);
```
3、日志处理:逐行读取日志文件,进行数据分析或统计。
```php
$file = fopen("access.log", "r");
while ($line = fgets($file)) {
$data = parseLogLine($line);
// 处理日志数据
}
fclose($file);
```
4、数据导入导出:处理数据库的大批量数据导入导出,使用分批处理策略。
```php
$batchSize = 1000;
$offset = 0;
while (true) {
$data = fetchDataFromDatabase($batchSize, $offset);
if (empty($data)) {
break;
}
// 处理数据
$offset += $batchSize;
}
```
性能优化
1、缓存机制:利用缓存技术减少重复读取文件的操作。
```php
$cache = new Redis();
$fileKey = "file_cache";
if ($cache->exists($fileKey)) {
$data = $cache->get($fileKey);
} else {
$data = readFileContents("largefile.txt");
$cache->set($fileKey, $data);
}
```
2、多线程处理:使用PHP的多线程扩展,如pthreads,并行处理文件。
```php
class FileProcessor extends Thread {
private $file;
public function __construct($file) {
$this->file = $file;
}
public function run() {
// 处理文件
}
}
$threads = [];
foreach ($files as $file) {
$thread = new FileProcessor($file);
$thread->start();
$threads[] = $thread;
}
foreach ($threads as $thread) {
$thread->join();
}
```
3、硬件优化:提升服务器硬件性能,如增加内存、使用SSD硬盘等。
安全考虑
1、文件验证:在上传文件前进行类型和大小验证,防止恶意文件上传。
```php
$allowedTypes = ['text/plain', 'application/pdf'];
$maxSize = 1024 * 1024 * 100; // 100MB
if (!in_array($_FILES['file']['type'], $allowedTypes) || $_FILES['file']['size'] > $maxSize) {
die("Invalid file");
}
```
2、权限控制:合理设置文件和目录的权限,避免未授权访问。
```php
chmod("largefile.txt", 0644);
```
3、错误处理:妥善处理文件操作中的异常和错误,防止信息泄露。
```php
try {
$file = fopen("largefile.txt", "r");
// 处理文件
} catch (Exception $e) {
error_log($e->getMessage());
die("File processing error");
}
```
PHP大文件处理是一个复杂且多面的任务,需要综合考虑性能、资源、安全和实际应用场景,通过合理选择处理策略、优化代码和配置、以及注意安全细节,可以有效提升大文件处理的效率和稳定性,希望本文的探讨能为广大PHP开发者提供有益的参考和启示。
关键词:PHP大文件处理, 分块读取, 流式处理, PHP配置, 临时文件, 异步处理, 文件上传, 断点续传, 文件下载, 日志处理, 数据导入导出, 缓存机制, 多线程处理, 硬件优化, 文件验证, 权限控制, 错误处理, ReactPHP, Redis, pthreads, 性能优化, 安全考虑, 内存消耗, 执行时间, I/O性能, 网络传输, Web开发, PHP编程, 文件操作, 文件分割, 文件合并, 数据处理, 服务器性能, 文件类型验证, 文件大小验证, 恶意文件上传, 文件权限, 异常处理, 信息泄露, 代码优化, 实践应用, 开发者参考







本文标签属性:
PHP大文件处理:php打开大文件