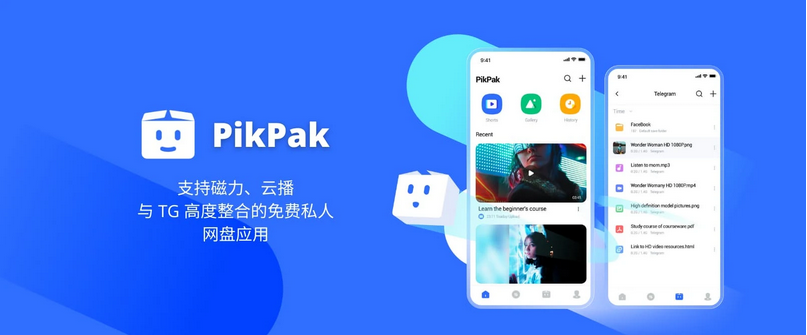
推荐阅读:
[AI-人工智能]免翻墙的AI利器:樱桃茶·智域GPT,让你轻松使用ChatGPT和Midjourney - 免费AIGC工具 - 拼车/合租账号 八折优惠码: AIGCJOEDISCOUNT2024
[AI-人工智能]银河录像局: 国内可靠的AI工具与流媒体的合租平台 高效省钱、现号秒发、翻车赔偿、无限续费|95折优惠码: AIGCJOE
[AI-人工智能]免梯免翻墙-ChatGPT拼车站月卡 | 可用GPT4/GPT4o/o1-preview | 会话隔离 | 全网最低价独享体验ChatGPT/Claude会员服务
[AI-人工智能]边界AICHAT - 超级永久终身会员激活 史诗级神器,口碑炸裂!300万人都在用的AI平台
PHP设计模式是提升Linux环境下代码质量和可维护性的关键工具。通过应用各种设计模式,如单例、工厂、观察者等,开发者能构建出结构清晰、易于扩展和维护的代码。这不仅提高了开发效率,还降低了后期维护成本。掌握PHP设计模式对于通过技术面试、提升职业竞争力至关重要。本文将探讨PHP设计模式的核心概念、常见模式及其在实际项目中的应用,助力开发者写出更优质的PHP代码。
本文目录导读:
在软件开发领域,设计模式是一种被广泛认可的最佳实践,它提供了一种解决常见问题的通用解决方案,PHP作为一种流行的编程语言,同样受益于设计模式的应用,本文将深入探讨PHP设计模式的概念、分类及其在实际开发中的应用,帮助开发者提升代码质量和可维护性。
设计模式概述
设计模式(Design Pattern)最早由Erich Gamma、Richard Helm、Ralph JohnsOn和John Vlissides四人提出,并在他们的著作《设计模式:可复用面向对象软件的基础》中详细阐述,设计模式主要分为三类:创建型模式、结构型模式和行为型模式。
1、创建型模式:关注对象的创建过程,主要包括单例模式、工厂模式、抽象工厂模式、建造者模式和原型模式。
2、结构型模式:关注类和对象之间的组合,主要包括适配器模式、桥接模式、装饰器模式、组合模式、外观模式、享元模式和代理模式。
3、行为型模式:关注对象之间的通信,主要包括责任链模式、命令模式、解释器模式、迭代器模式、中介者模式、备忘录模式、观察者模式、状态模式、策略模式、模板方法模式和访问者模式。
PHP中的创建型模式
1、单例模式:确保一个类只有一个实例,并提供一个全局访问点,在PHP中,单例模式常用于数据库连接等场景。
```php
class Database {
private static $instance = null;
private funCTIon __construct() {}
public static function getInstance() {
if (self::$instance === null) {
self::$instance = new Database();
}
return self::$instance;
}
private function __clone() {}
private function __wakeup() {}
}
```
2、工厂模式:定义一个用于创建对象的接口,让子类决定实例化哪一个类,工厂模式在PHP中常用于对象的创建和管理。
```php
interface Product {
public function getName();
}
class Book implements Product {
public function getName() {
return "Book";
}
}
class Factory {
public static function createProduct($type) {
switch ($type) {
case 'book':
return new Book();
default:
throw new Exception("Unknown product type.");
}
}
}
```
3、抽象工厂模式:提供一个接口,用于创建相关或依赖对象的家族,而不需要指定具体类。
```php
interface AbstractFactory {
public function createProductA();
public function createProductB();
}
class ConcreteFactory1 implements AbstractFactory {
public function createProductA() {
return new ProductA1();
}
public function createProductB() {
return new ProductB1();
}
}
```
4、建造者模式:将一个复杂对象的构建与它的表示分离,使得同样的构建过程可以创建不同的表示。
```php
class Product {
private $parts = [];
public function addPart($part) {
$this->parts[] = $part;
}
public function show() {
echo "Product Parts: " . implode(', ', $this->parts) . "
";
}
}
interface Builder {
public function buildPartA();
public function buildPartB();
public function getResult();
}
class ConcreteBuilder implements Builder {
private $product;
public function __construct() {
$this->product = new Product();
}
public function buildPartA() {
$this->product->addPart('PartA');
}
public function buildPartB() {
$this->product->addPart('PartB');
}
public function getResult() {
return $this->product;
}
}
```
5、原型模式:通过复制现有的实例来创建新的实例,而不是通过构造函数。
```php
class Prototype {
private $property;
public function __construct($property) {
$this->property = $property;
}
public function __clone() {
$this->property = clone $this->property;
}
}
```
PHP中的结构型模式
1、适配器模式:将一个类的接口转换成客户期望的另一个接口,使得原本接口不兼容的类可以一起工作。
```php
interface Target {
public function request();
}
class Adaptee {
public function specificRequest() {
return "Specific request";
}
}
class Adapter implements Target {
private $adaptee;
public function __construct(Adaptee $adaptee) {
$this->adaptee = $adaptee;
}
public function request() {
return $this->adaptee->specificRequest();
}
}
```
2、桥接模式:将抽象部分与实现部分分离,使它们可以独立地变化。
```php
interface Implementor {
public function operation();
}
class ConcreteImplementorA implements Implementor {
public function operation() {
return "ConcreteImplementorA operation";
}
}
abstract class Abstraction {
protected $implementor;
public function __construct(Implementor $implementor) {
$this->implementor = $implementor;
}
abstract public function operation();
}
class RefinedAbstraction extends Abstraction {
public function operation() {
return "RefinedAbstraction: " . $this->implementor->operation();
}
}
```
3、装饰器模式:动态地给一个对象添加一些额外的职责,而不改变其接口。
```php
interface Component {
public function operation();
}
class ConcreteComponent implements Component {
public function operation() {
return "ConcreteComponent operation";
}
}
class Decorator implements Component {
protected $component;
public function __construct(Component $component) {
$this->component = $component;
}
public function operation() {
return $this->component->operation();
}
}
class ConcreteDecoratorA extends Decorator {
public function operation() {
return "ConcreteDecoratorA(" . parent::operation() . ")";
}
}
```
4、组合模式:将对象组合成树形结构以表示“部分-整体”的层次结构,使得客户可以统一使用单个对象和组合对象。
```php
interface Component {
public function operation();
}
class Leaf implements Component {
public function operation() {
return "Leaf operation";
}
}
class Composite implements Component {
private $children = [];
public function add(Component $component) {
$this->children[] = $component;
}
public function operation() {
$result = "Composite operation";
foreach ($this->children as $child) {
$result .= $child->operation();
}
return $result;
}
}
```
5、外观模式:为子系统中的一组接口提供一个一致的界面,使得子系统更容易使用。
```php
class SubsystemA {
public function operationA() {
return "SubsystemA operation";
}
}
class SubsystemB {
public function operationB() {
return "SubsystemB operation";
}
}
class Facade {
private $subsystemA;
private $subsystemB;
public function __construct() {
$this->subsystemA = new SubsystemA();
$this->subsystemB = new SubsystemB();
}
public function operation() {
return $this->subsystemA->operationA() . " " . $this->subsystemB->operationB();
}
}
```
PHP中的行为型模式
1、责任链模式:使多个对象都有机会处理请求,从而避免请求的发送者和接收者之间的耦合关系。
```php
interface Handler {
public function handle($request);
public function setNext(Handler $handler);
}
class ConcreteHandlerA implements Handler {
private $nextHandler;
public function handle($request) {
if ($request === 'A') {
return "Handled by ConcreteHandlerA";
} elseif ($this->nextHandler !== null) {
return $this->nextHandler->handle($request);
}
return "No handler for request";
}
public function setNext(Handler $handler) {
$this->nextHandler = $handler;
}
}
class ConcreteHandlerB implements Handler {
private $nextHandler;
public function handle($request) {
if ($request === 'B') {
return "Handled by ConcreteHandlerB";
} elseif ($this->nextHandler !== null) {
return $this->nextHandler->handle($request);
}
return "No handler for request";
}
public function setNext(Handler $handler) {
$this->nextHandler = $handler;
}
}
```
2、命令模式:将请求封装为一个对象,从而可以使用不同的请求、队列或日志来参数化其他对象。
```php
interface Command {
public function execute();
}
class ConcreteCommand implements Command {
private $receiver;
public function __construct(







本文标签属性:
PHP设计模式:php设计模式及使用场景