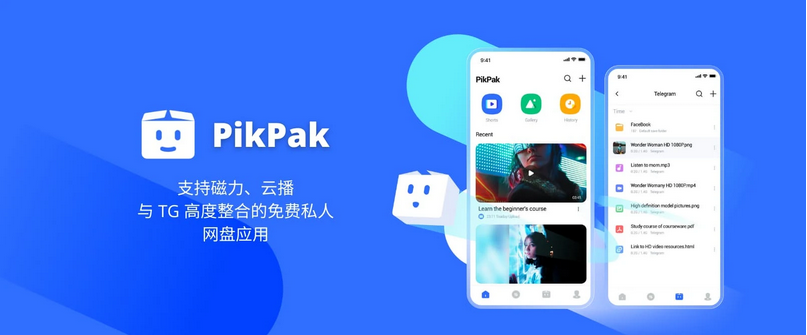
推荐阅读:
[AI-人工智能]免翻墙的AI利器:樱桃茶·智域GPT,让你轻松使用ChatGPT和Midjourney - 免费AIGC工具 - 拼车/合租账号 八折优惠码: AIGCJOEDISCOUNT2024
[AI-人工智能]银河录像局: 国内可靠的AI工具与流媒体的合租平台 高效省钱、现号秒发、翻车赔偿、无限续费|95折优惠码: AIGCJOE
[AI-人工智能]免梯免翻墙-ChatGPT拼车站月卡 | 可用GPT4/GPT4o/o1-preview | 会话隔离 | 全网最低价独享体验ChatGPT/Claude会员服务
[AI-人工智能]边界AICHAT - 超级永久终身会员激活 史诗级神器,口碑炸裂!300万人都在用的AI平台
本文探讨了Linux操作系统下PHP桥接模式的应用与实践。桥接设计模式旨在将抽象部分与实现部分分离,使它们可以独立变化。通过详细解析PHP桥接模式的概念与实现,文章展示了其在实际项目中的优势,为开发者提供了灵活性和扩展性。
本文目录导读:
随着信息技术的快速发展,软件架构设计在软件开发中变得越来越重要,在众多设计模式中,桥接模式(Bridge Pattern)是一种常用的结构型设计模式,它通过将抽象与其实现解耦,使得抽象和实现可以独立变化,本文将详细介绍PHP桥接模式的概念、原理及其在实际项目中的应用。
PHP桥接模式的概念
桥接模式是一种将抽象部分与实现部分分离的设计模式,使得它们可以独立地变化,在PHP中,桥接模式主要通过接口和抽象类来实现,它包括以下四个角色:
1、抽象类(Abstraction):定义抽象类的接口,包含一个实现类的引用。
2、具体抽象类(RefinedAbstraction):继承抽象类,并添加新的具体操作。
3、实现类(Implementor):定义实现类的接口,用于实现抽象类中的操作。
4、具体实现类(ConcreteImplementor):继承实现类,并实现具体的操作。
PHP桥接模式的原理
桥接模式的原理可以通过以下四个步骤来概括:
1、定义抽象类和实现类:抽象类用于定义抽象的方法和属性,实现类用于实现抽象类中的方法。
2、在抽象类中添加一个实现类的引用:这样,抽象类就可以通过这个引用来调用实现类的方法。
3、定义具体抽象类和具体实现类:具体抽象类继承抽象类,并添加新的具体操作;具体实现类继承实现类,并实现具体的操作。
4、实现类的变化不影响抽象类:由于抽象类和实现类之间是通过引用关联的,所以实现类的变化不会影响到抽象类。
PHP桥接模式的应用
下面通过一个简单的例子来展示PHP桥接模式的应用。
场景:一个图形库,需要支持多种图形的绘制,包括圆形、矩形等,每种图形都有不同的绘制方式,如使用不同的画笔。
1、定义抽象类和实现类
interface DrawAPI { public function drawCircle($radius, $x, $y); public function drawRectangle($width, $height, $x, $y); } class RedCircle implements DrawAPI { public function drawCircle($radius, $x, $y) { echo "Drawing a red circle at ($x, $y) with radius $radius "; } public function drawRectangle($width, $height, $x, $y) { echo "Drawing a red rectangle at ($x, $y) with width $width and height $height "; } } class GreenRectangle implements DrawAPI { public function drawCircle($radius, $x, $y) { echo "Drawing a green circle at ($x, $y) with radius $radius "; } public function drawRectangle($width, $height, $x, $y) { echo "Drawing a green rectangle at ($x, $y) with width $width and height $height "; } }
2、定义具体抽象类
abstract class Shape { protected $drawAPI; public function __construct(DrawAPI $drawAPI) { $this->drawAPI = $drawAPI; } abstract public function draw(); } class Circle extends Shape { private $radius; private $x; private $y; public function __construct(DrawAPI $drawAPI, $radius, $x, $y) { parent::__construct($drawAPI); $this->radius = $radius; $this->x = $x; $this->y = $y; } public function draw() { $this->drawAPI->drawCircle($this->radius, $this->x, $this->y); } } class Rectangle extends Shape { private $width; private $height; private $x; private $y; public function __construct(DrawAPI $drawAPI, $width, $height, $x, $y) { parent::__construct($drawAPI); $this->width = $width; $this->height = $height; $this->x = $x; $this->y = $y; } public function draw() { $this->drawAPI->drawRectangle($this->width, $this->height, $this->x, $this->y); } }
3、实现具体实现类
$redCircle = new Circle(new RedCircle(), 10, 20, 30); $redRectangle = new Rectangle(new RedCircle(), 20, 30, 40, 50); $greenCircle = new Circle(new GreenRectangle(), 10, 20, 30); $greenRectangle = new Rectangle(new GreenRectangle(), 20, 30, 40, 50);
4、实现类的变化不影响抽象类
当我们需要更换画笔时,只需创建一个新的实现类,而不需要修改抽象类和具体抽象类,创建一个新的画笔类BluePen
,并实现DrawAPI
接口。
class BluePen implements DrawAPI { public function drawCircle($radius, $x, $y) { echo "Drawing a blue circle at ($x, $y) with radius $radius "; } public function drawRectangle($width, $height, $x, $y) { echo "Drawing a blue rectangle at ($x, $y) with width $width and height $height "; } }
我们可以创建一个使用BluePen
的圆形对象:
$blueCircle = new Circle(new BluePen(), 10, 20, 30);
PHP桥接模式通过将抽象与实现分离,使得抽象和实现可以独立变化,在实际项目中,桥接模式可以帮助我们更好地管理复杂的类层次结构,提高代码的可复用性和可维护性,通过本文的介绍,我们了解了PHP桥接模式的概念、原理及其应用,希望对大家在实际项目中的应用有所帮助。
关键词:PHP, 桥接模式, 设计模式, 抽象类, 实现类, 接口, 解耦, 抽象与实现分离, 可复用性, 可维护性, 图形库, 画笔, 圆形, 矩形, 场景, 类层次结构, 管理复杂度, 灵活扩展, 实际应用, 代码优化, 软件架构, 开发技巧, 编程实践, 设计原则, 软件设计, 代码重构, 软件工程, 设计理念, 软件开发, 软件设计模式, PHP编程, PHP设计模式, PHP架构, PHP开发, PHP实战, PHP技巧, PHP框架, PHP应用, PHP优化, PHP编程技巧, PHP设计思想, PHP代码优化, PHP架构设计, PHP设计理念, PHP编程规范, PHP开发经验, PHP设计模式实践, PHP架构实践, PHP开发技巧, PHP编程心得, PHP设计心得, PHP架构心得, PHP编程之道, PHP设计之道, PHP架构之道







本文标签属性:
PHP桥接模式:桥接模式csdn
设计模式实践:设计模式实例剖析与深入解读