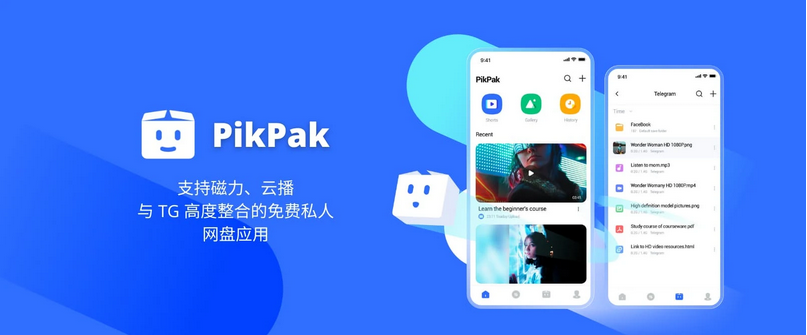
推荐阅读:
[AI-人工智能]免翻墙的AI利器:樱桃茶·智域GPT,让你轻松使用ChatGPT和Midjourney - 免费AIGC工具 - 拼车/合租账号 八折优惠码: AIGCJOEDISCOUNT2024
[AI-人工智能]银河录像局: 国内可靠的AI工具与流媒体的合租平台 高效省钱、现号秒发、翻车赔偿、无限续费|95折优惠码: AIGCJOE
[AI-人工智能]免梯免翻墙-ChatGPT拼车站月卡 | 可用GPT4/GPT4o/o1-preview | 会话隔离 | 全网最低价独享体验ChatGPT/Claude会员服务
[AI-人工智能]边界AICHAT - 超级永久终身会员激活 史诗级神器,口碑炸裂!300万人都在用的AI平台
PHP设计模式是提升Linux环境下代码质量和可维护性的重要工具。常见的设计模式包括单例模式、工厂模式、策略模式等,它们通过规范代码结构,增强模块化和复用性,降低耦合度。合理应用PHP设计模式,能有效提高开发效率,简化代码维护,使项目更健壮、灵活。掌握这些模式,对PHP开发者至关重要,有助于构建高质量、易管理的Linux应用程序。
本文目录导读:
在软件开发领域,设计模式是一种被广泛认可和使用的解决方案,用于解决特定类型的问题,PHP作为一种流行的编程语言,同样受益于设计模式的应用,本文将深入探讨PHP设计模式的概念、分类及其在实际开发中的应用,帮助开发者提升代码质量和可维护性。
设计模式概述
设计模式(Design Pattern)是在软件工程中总结出的一套经典解决方案,它们描述了在特定环境下解决常见问题的方法,设计模式的目的是提高代码的可重用性、可读性和可维护性,设计模式通常分为三类:创建型模式、结构型模式和行为型模式。
创建型模式
创建型模式主要关注对象的创建过程,提供了一种灵活的方式来创建对象,使得对象的创建与使用分离。
1、单例模式(Singleton)
单例模式确保一个类只有一个实例,并提供一个全局访问点,在PHP中,单例模式常用于数据库连接、配置管理等场景。
```php
class Singleton {
private static $instance = null;
private function __construct() {}
public static function getInstance() {
if (self::$instance === null) {
self::$instance = new Singleton();
}
return self::$instance;
}
private function __clone() {}
private function __wakeup() {}
}
```
2、工厂模式(Factory)
工厂模式定义一个用于创建对象的接口,让子类决定实例化哪一个类,工厂模式适用于对象创建逻辑复杂或需要根据不同条件创建不同对象的情况。
```php
interface Product {
public function operation();
}
class ConcreteProductA implements Product {
public function operation() {
echo "Product A operation
";
}
}
class ConcreteProductB implements Product {
public function operation() {
echo "Product B operation
";
}
}
class Factory {
public static function createProduct($type) {
switch ($type) {
case 'A':
return new ConcreteProductA();
case 'B':
return new ConcreteProductB();
default:
throw new Exception("Unknown product type");
}
}
}
```
3、抽象工厂模式(Abstract Factory)
抽象工厂模式提供一个接口,用于创建相关或依赖对象的家族,而不需要指定具体类,适用于需要创建一系列相关对象的情况。
```php
interface AbstractFactory {
public function createProductA();
public function createProductB();
}
class ConcreteFactory1 implements AbstractFactory {
public function createProductA() {
return new ConcreteProductA1();
}
public function createProductB() {
return new ConcreteProductB1();
}
}
class ConcreteFactory2 implements AbstractFactory {
public function createProductA() {
return new ConcreteProductA2();
}
public function createProductB() {
return new ConcreteProductB2();
}
}
```
结构型模式
结构型模式关注类和对象之间的组合,用于设计更灵活、更具可扩展性的结构。
1、适配器模式(Adapter)
适配器模式将一个类的接口转换成客户期望的另一个接口,使得原本接口不兼容的类可以一起工作。
```php
interface Target {
public function request();
}
class Adaptee {
public function specificRequest() {
echo "Adaptee specific request
";
}
}
class Adapter implements Target {
private $adaptee;
public function __construct(Adaptee $adaptee) {
$this->adaptee = $adaptee;
}
public function request() {
$this->adaptee->specificRequest();
}
}
```
2、装饰器模式(Decorator)
装饰器模式动态地给一个对象添加一些额外的职责,而不改变其接口,适用于需要扩展对象功能的情况。
```php
interface Component {
public function operation();
}
class ConcreteComponent implements Component {
public function operation() {
echo "ConcreteComponent operation
";
}
}
class Decorator implements Component {
protected $component;
public function __construct(Component $component) {
$this->component = $component;
}
public function operation() {
$this->component->operation();
}
}
class ConcreteDecoratorA extends Decorator {
public function operation() {
parent::operation();
echo "ConcreteDecoratorA operation
";
}
}
```
3、代理模式(Proxy)
代理模式为其他对象提供一种代理以控制对这个对象的访问,适用于需要控制对象访问权限或延迟初始化的情况。
```php
interface Subject {
public function request();
}
class RealSubject implements Subject {
public function request() {
echo "RealSubject request
";
}
}
class Proxy implements Subject {
private $realSubject;
public function request() {
if ($this->realSubject === null) {
$this->realSubject = new RealSubject();
}
$this->realSubject->request();
}
}
```
行为型模式
行为型模式关注对象之间的通信,用于定义对象间如何交互以及如何分配职责。
1、观察者模式(Observer)
观察者模式定义对象间的一种一对多依赖关系,当一个对象状态改变时,所有依赖于它的对象都会得到通知并自动更新。
```php
interface Observer {
public function update($subject);
}
interface Subject {
public function attach(Observer $observer);
public function detach(Observer $observer);
public function notify();
}
class ConcreteSubject implements Subject {
private $observers = [];
private $state;
public function attach(Observer $observer) {
$this->observers[] = $observer;
}
public function detach(Observer $observer) {
$key = array_search($observer, $this->observers, true);
if ($key !== false) {
unset($this->observers[$key]);
}
}
public function notify() {
foreach ($this->observers as $observer) {
$observer->update($this);
}
}
public function setState($state) {
$this->state = $state;
$this->notify();
}
public function getState() {
return $this->state;
}
}
class ConcreteObserverA implements Observer {
public function update($subject) {
echo "ConcreteObserverA: Reacted to the event.
";
}
}
```
2、策略模式(Strategy)
策略模式定义一系列算法,将每个算法封装起来,并使它们可以互相替换,策略模式使算法的变化独立于使用算法的客户。
```php
interface Strategy {
public function execute($data);
}
class ConcreteStrategyA implements Strategy {
public function execute($data) {
echo "ConcreteStrategyA: " . $data . "
";
}
}
class ConcreteStrategyB implements Strategy {
public function execute($data) {
echo "ConcreteStrategyB: " . $data . "
";
}
}
class Context {
private $strategy;
public function setStrategy(Strategy $strategy) {
$this->strategy = $strategy;
}
public function executeStrategy($data) {
$this->strategy->execute($data);
}
}
```
3、命令模式(Command)
命令模式将请求封装为一个对象,从而让你使用不同的请求、队列或日志请求来参数化其他对象,命令模式也支持可撤销的操作。
```php
interface Command {
public function execute();
}
class ConcreteCommand implements Command {
private $receiver;
public function __construct(Receiver $receiver) {
$this->receiver = $receiver;
}
public function execute() {
$this->receiver->action();
}
}
class Receiver {
public function action() {
echo "Receiver action
";
}
}
class Invoker {
private $command;
public function setCommand(Command $command) {
$this->command = $command;
}
public function executeCommand() {
$this->command->execute();
}
}
```
PHP设计模式是提升代码质量和可维护性的重要工具,通过合理应用设计模式,开发者可以编写出更灵活、更易扩展和更易维护的代码,本文介绍了常见的PHP设计模式及其应用示例,希望能为PHP开发者在实际项目中应用设计模式提供参考。
关键词
PHP设计模式, 单例模式, 工厂模式, 抽象工厂模式, 适配器模式, 装饰器模式, 代理模式, 观察者模式, 策略模式, 命令模式, 创建型模式, 结构型模式, 行为型模式, 对象创建, 接口转换, 功能扩展, 访问控制, 依赖关系, 算法封装, 请求封装, 可维护性, 可重用性, 可读性, 软件工程, 解决方案, 类与对象, 组合, 通信, 职责分配, 实例







本文标签属性:
PHP设计模式:Php设计模式有哪些